Cancel Video Chat - From Lobby
If a customer was to click the ‘cancel video chat’ button it should navigate them back to this initial
support screen.
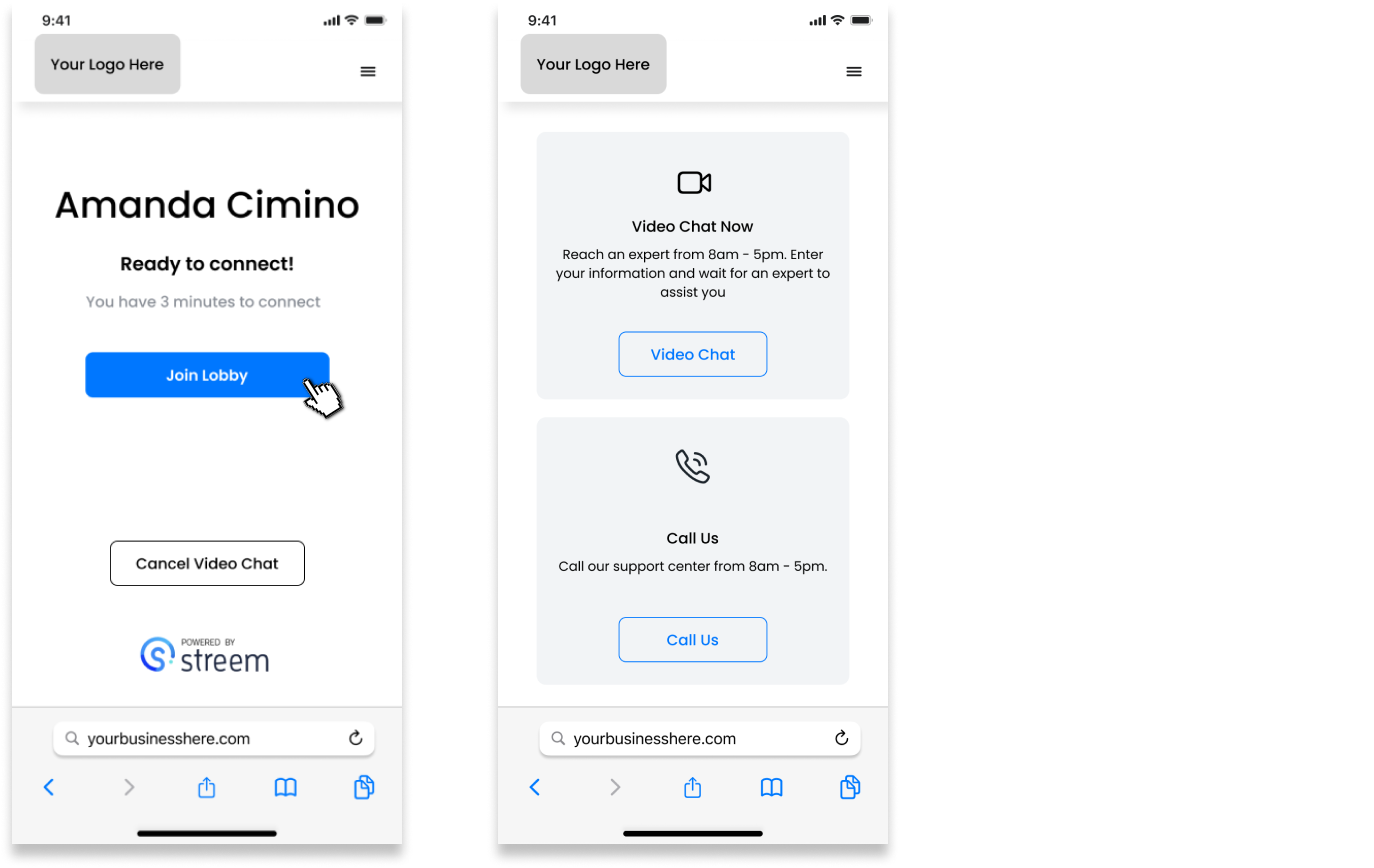
Code snippets from the example app
const handleCancel = async () => {
try {
await fetch(`${serverConfig.endpoint}/groups/AGENT/reservations/${reservationSid}/cancel`, {
method: 'POST'
});
history.push(`/`);
} catch (e) {
console.log(e);
}
};
router.post('/:groupName/reservations/:reservationSid/cancel',
async (req: Request, res: Response) => {
const { groupName, reservationSid } = req.params;
await streemApi.cancelReservation(streemConfig.companySid, groupName, reservationSid);
res.json(await streemApi.getReservation(streemConfig.companySid, groupName, reservationSid));
},
);
async cancelReservation(
companySid: string,
groupName: string,
reservationSid: string,
): Promise<void> {
const url = `${this.baseUrl}/v1/companies/${companySid}/groups/${groupName}/reservations/${reservationSid}/cancel`;
await axios.post<{ group_reservation: GroupReservation }>(url, {}, { ...this.config });
}
Updated over 1 year ago
What’s Next