Join Lobby
Once the queue is up customer are taken to this screen. This screen let’s them know the expert’s name that’s ready to connect and that they have 3 minutes to join the call. Once the customer clicks ‘join lobby’ they are taken into the Streem experience and the first screen they land on in Streem’s terms and conditions.
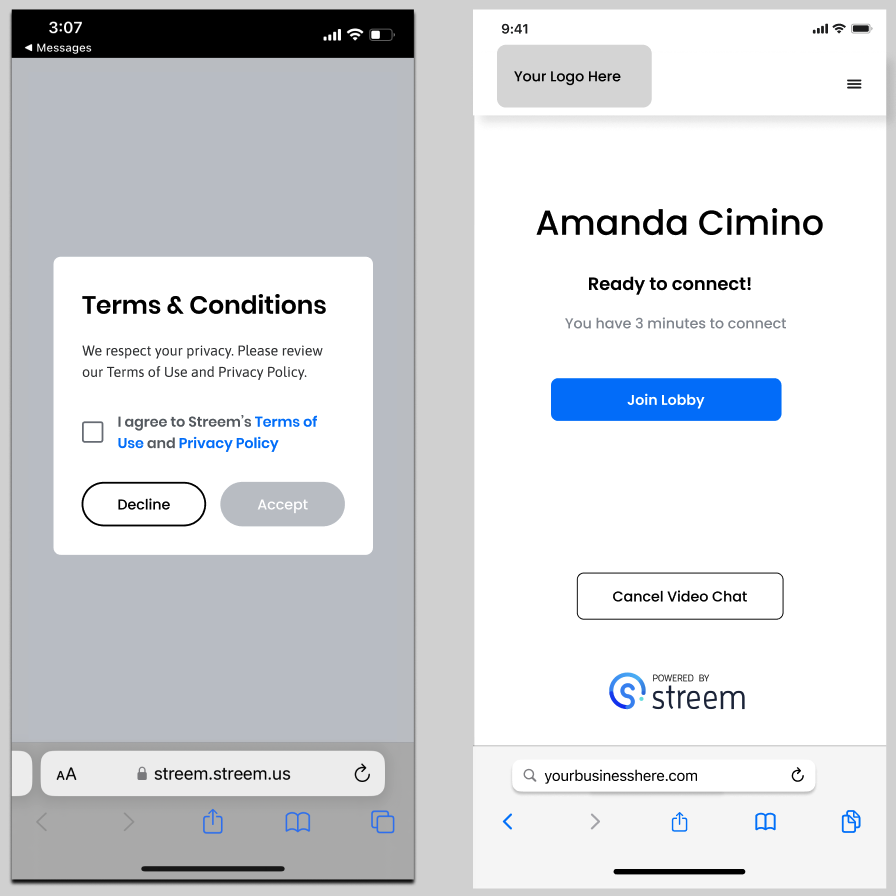
Code snippets from the example app
useEffect(() => {
const getJoinUrl = async () => {
try {
const response = await fetch(`${serverConfig.endpoint}/groups/${GroupName.DEFAULT}/reservations/${reservationSid}/join-url`, {
method: 'POST',
headers: { 'Content-Type': 'application/json' }
});
const joinUrl = await response.json();
setJoinUrl(joinUrl['join_url']);
} catch(e) {
console.error(e);
}
}
getJoinUrl().catch((e) => console.error(e));
}, [reservationSid]);
const handleJoin = () => {
if (joinUrl) {
window.location.href = joinUrl;
}
};
import Streem from '@streem/sdk-node';
...
router.post(
'/:groupName/reservations/:reservationSid/join-url',
async (req: Request, res: Response) => {
const { reservationSid } = req.params;
const { externalUserId } = req.body;
Streem.init(streemConfig.apiKeyId, streemConfig.apiKeySecret, `${streemConfig.apiEnv}`);
const tokenBuilder = new Streem.TokenBuilder();
tokenBuilder.userId = externalUserId;
tokenBuilder.name = 'Click To Video Example';
tokenBuilder.reservationSid = reservationSid;
const token = await tokenBuilder.build();
res.json({ join_url: `https://${streemConfig.companyCode}.cv.${streemConfig.apiEnv}.streem.cloud/token#token=${token}` });
},
);
Updated almost 2 years ago
What’s Next