Intake Form
After a user clicks the video chat button they should be taken to an intake form. Here you gather
information about the customer and the issue they’re experiencing. This information will be passed over
to the Streem experts (link out to expert page) so before they enter the call they know issues and
pain points their customers are experiencing.
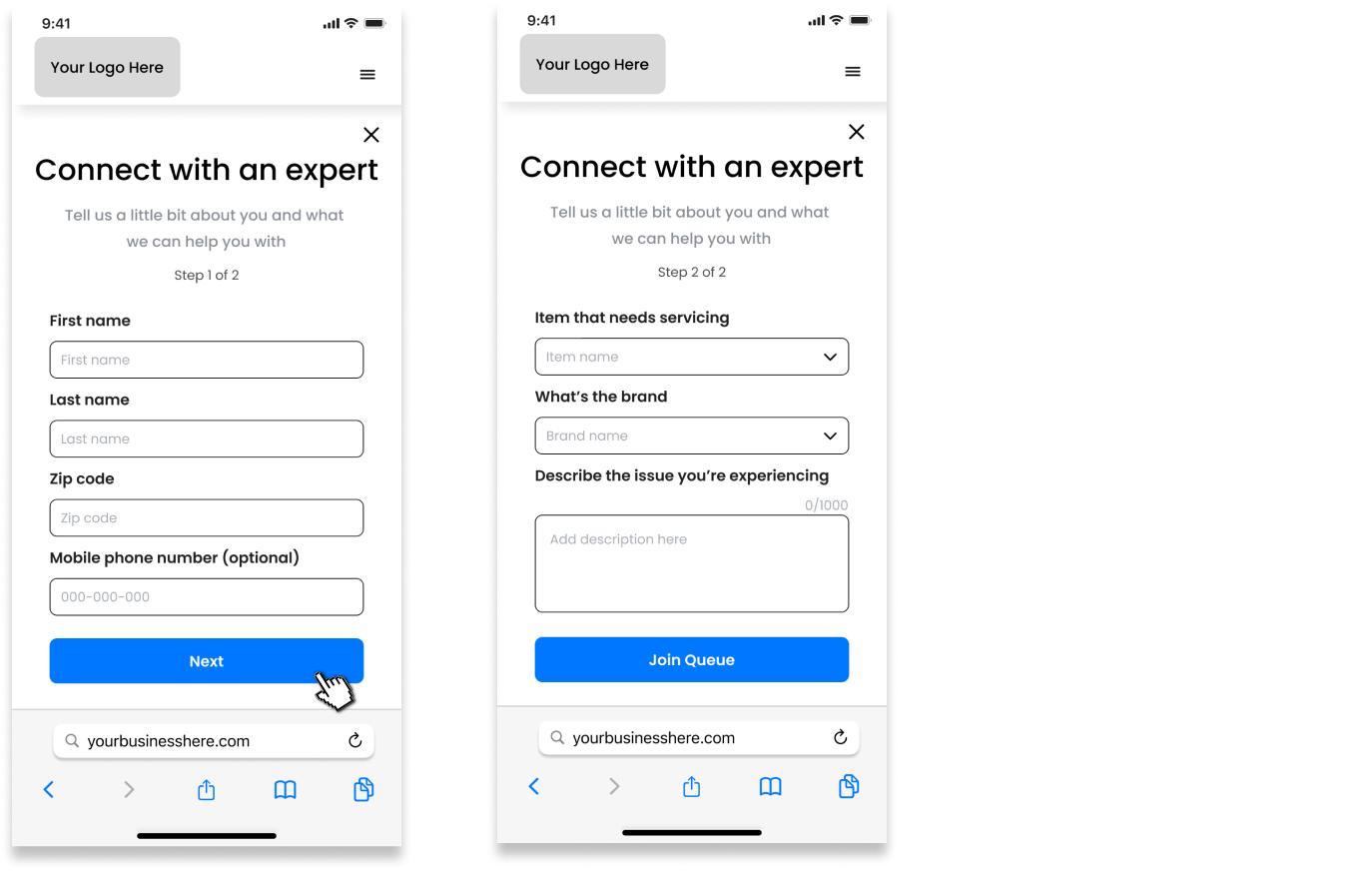
Code snippets from the example app
const handleSubmit = async (e: FormEvent) => {
e.preventDefault();
const response = await fetch(`${serverConfig.endpoint}/groups/AGENT/reservations`, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
externalUserId: email,
details: [
{ label: 'name', value: name},
{ label: 'item', value: item },
{ label: 'description', value: description }
]
}),
});
const reservation = await response.json();
history.push(`/hold/r/${reservation['reservation_sid']}`);
};
router.post('/:groupName/reservations', async (req: CreateReservationRequest, res: Response) => {
const { groupName } = req.params;
const { externalUserId, details } = req.body;
const reservation = await streemApi.createReservation(
streemConfig.companySid,
groupName,
externalUserId,
details,
);
res.json(reservation);
});
async createReservation(
companySid: string,
groupName: string,
externalUserId: string,
details: { label: string; value: string }[],
): Promise<GroupReservation> {
const url = `${this.baseUrl}/v1/companies/${companySid}/groups/${groupName}/reservations`;
const res = await axios.post<{ group_reservation: GroupReservation }>(
url,
{
externalUserId: externalUserId,
queue: true,
reserve_for_seconds: 60,
details: [{ label: 'Source', value: 'ClickToVideoExample' }, ...details],
},
{ ...this.config },
);
return res.data.group_reservation;
}
Updated over 1 year ago
What’s Next