The Queue
From the moment the customer taps ‘start video chat’ they enter the ‘queue’ screen. In this example
you let the customer know they are now in the queue and can give them an estimated wait time.
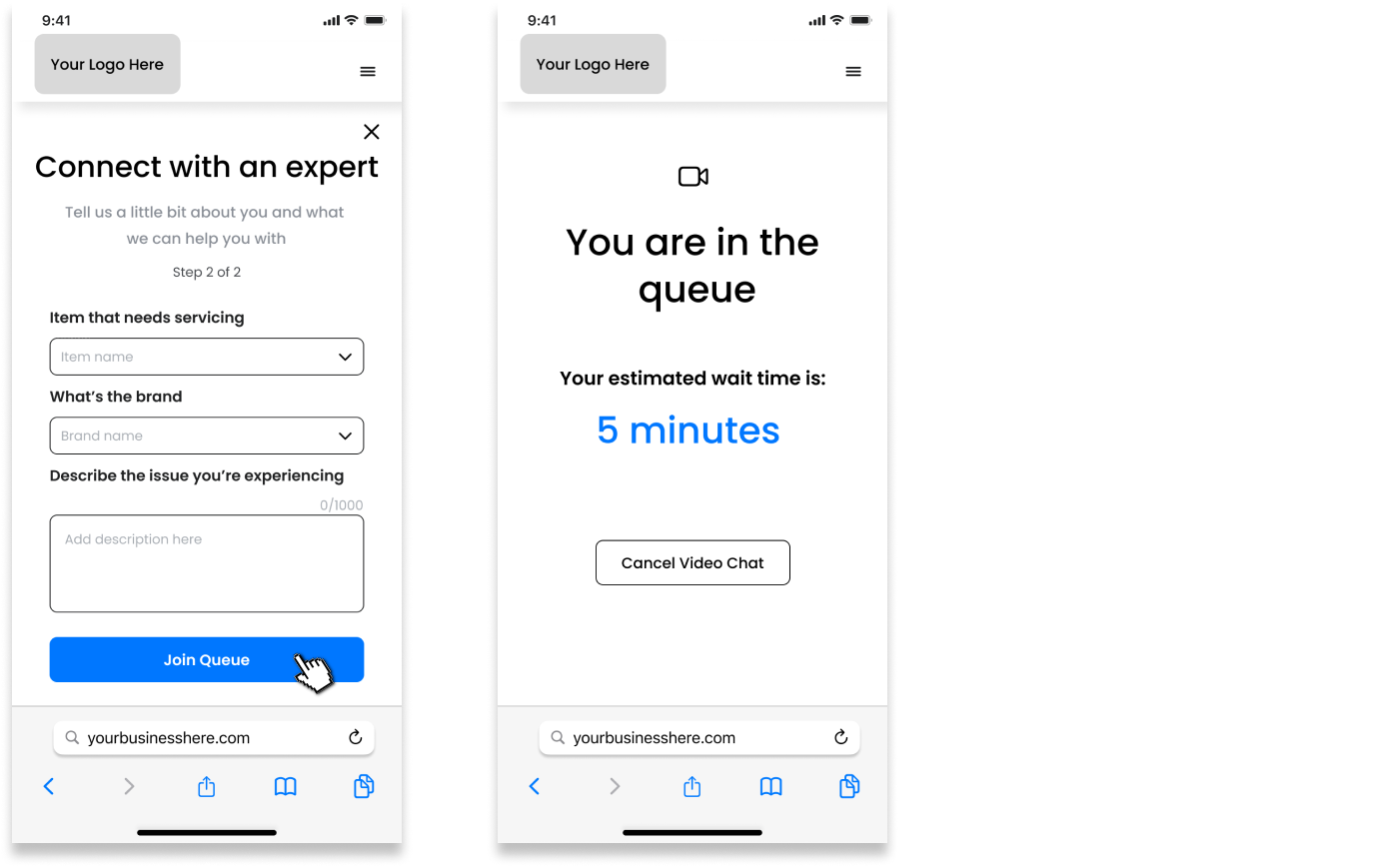
- Note: Estimate wait time is based on an aggregation of the wait time from previous calls. If you have never completed a call, the API will return
null
for theestimated_wait_until
field.
Code snippets from the example app
useEffect(() => {
const eventSource = new EventSource(`${serverConfig.endpoint}/events/g/${GroupName.DEFAULT}/r/${reservationSid}`);
eventSource.onmessage = (event) => {
const reservation = JSON.parse(event.data);
console.log("Hold page: ", reservation);
setReservationStatus(reservation['reservation_status']);
setQueuePosition(reservation['queue_position']);
if (reservation['estimated_wait_until']) {
setInterval(() => {
setEstimatedWaitTime(Math.round((Date.parse(reservation['estimated_wait_until']) - Date.now()) / (60 * 1000)));
}, 5000);
}
};
}, [reservationSid, history]);
useEffect(() => {
switch(reservationStatus) {
case 'GROUP_RESERVATION_STATUS_MATCHED': {
history.push(`/join/r/${reservationSid}`);
break;
}
case 'GROUP_RESERVATION_STATUS_EXPIRED': {
history.push(`/rejoin/r/${reservationSid}`);
break;
}
case 'GROUP_RESERVATION_STATUS_CANCELED': {
history.push(`/`);
break;
}
}
}, [reservationStatus, reservationSid, history]);
export function clientEventsHandler (req: Request, res: Response) {
const { reservationSid } = req.params;
const newClientEventResponse = {
reservationSid: reservationSid,
timestamp: Date.now(),
res
};
clientEventResponses.push(newClientEventResponse);
const headers = {
'Content-Type': 'text/event-stream',
'Connection': 'keep-alive',
'Cache-Control': 'no-cache'
};
newClientEventResponse.res.writeHead(200, headers);
newClientEventResponse.res.write(`data: ${JSON.stringify({})}\n\n`);
}
export function streemEventsHandler (req: Request, res: Response) {
const { event } = req.body;
if (event && event.event_type === 'group_reservation_updated') {
const updatedReservation = (event.payload as GroupReservationUpdated).updated_group_reservation;
clientEventResponses
.filter((clientEventResponse) => { return clientEventResponse.reservationSid === updatedReservation.reservation_sid })
.forEach((clientEventResponse) => {
clientEventResponse.res.write(`data: ${JSON.stringify(updatedReservation)}\n\n`);
});
}
res.status(200).end()
}
router.get('/:groupName/reservations/:reservationSid', async (req: Request, res: Response) => {
const { groupName, reservationSid } = req.params;
res.json(await streemApi.getReservation(streemConfig.companySid, groupName, reservationSid));
});
async getReservation(
companySid: string,
groupName: string,
reservationSid: string,
): Promise<GroupReservation> {
const url = `${this.baseUrl}/v1/companies/${companySid}/groups/${groupName}/reservations/${reservationSid}`;
const res = await axios<{ group_reservation: GroupReservation }>(url, { ...this.config });
return res.data.group_reservation;
}
Updated over 1 year ago
What’s Next